Almost all projects need to use some sort of icon. While using a simple icon in image format such as dropping a .png is simple at first, you will soon find it exhausting to find and add more image based icons later in app life cycle.
If you’re coming from the web development world, you would be pretty familiar with font icon libraries such as FontAwesome, Ionicons, etc. In this article we will try add font icon to NativeScript and use it easily with help of nativescript-fonticon
package.
Side note: There is another package called @nativescript-community/fonticon
but not so well maintained at the moment. So don’t be mistaking between the packages.
1. How it all works
Normally, all fonts you put in /src/fonts would be loaded automaticially. So actually, you can set the font-family
for your element such as <Label>, then directly input the character code which something like “\f098”. Which totally not make any sense for human readability, but it does work.
Basically nativescript-fonticon
package will scan for the provided .css file, lookup for all class name prefixed with the name you provided such as “fa-“, and remember its “content” which is the Unicode Character code present the actual icon in font file:
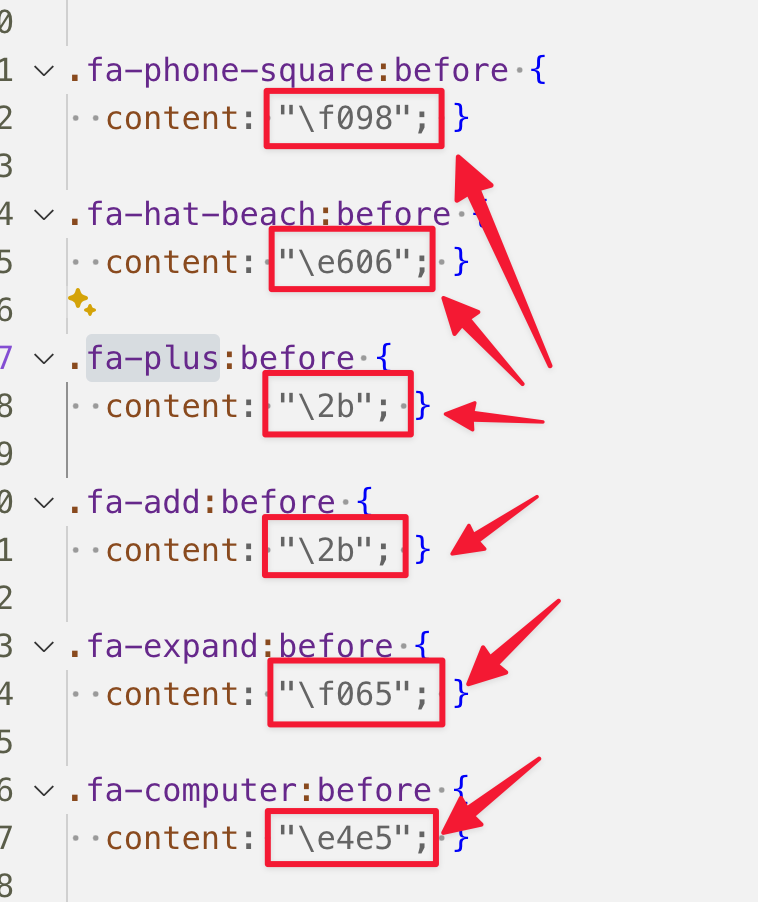
Then later it will build a map between normal class name of a font icon library of your choice to non-rememberable character code. So you can use the icon name in more memorable way such as: “fa-user”, “fa-computer”, etc. Cool!
2. Find the font icon library you want to use.
There is plenty of it. In this article, we will use the most beloved font icon set of the community: FontAwesome.
For downloading FontAwesome, you can go to: https://fontawesome.com/v6/download#v6-5-2
And grab your self a copy by clicking on Download web version. (This works same for Pro version of FontAwesome too)

In the downloaded zip package there will be .css and the font files itself. As mentioned earlier, if you are trying to use other font icon library, make sure that it does provide you with above two things or this setup won’t work.
3. Add font icon to NativeScript project
After have .css and font files ready, copy it into your project as following:
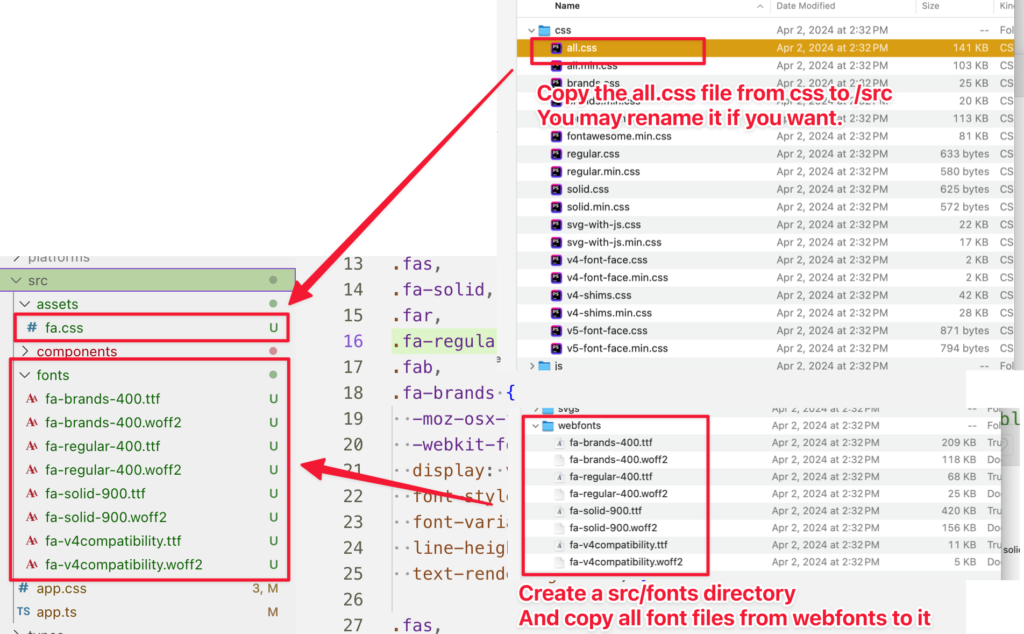
Somehow, .css will not work if you put it in root folder /src, but will works if you put in sub-directory such as /src/assets. So be careful.
4. Define classname & font-family
Next, you will have to define a class name, so you can easily override the font face of the element in template. For FontAwesome Free, add this to your app.css will do the trick:
.fa {
font-family: "Font Awesome 6 Free", "fa-regular-400";
font-style: normal;
font-weight: 400;
font-display: block;
}
.fas {
font-family: "Font Awesome 6 Free", "fa-solid-900";
font-style: normal;
font-weight: 900;
font-display: block;
}
Explain about font-family:
- “Font Awesome 6 Free” -> this is for iOS, because iOS will use the full name of font which you put in /src/fonts directory.
- “fa-solid-900” -> this is for Android, on Android side, it using the actual filename of the font you put in /src/fonts directory
- Read more at: https://docs.nativescript.org/project-structure/src/fonts
5. Config the nativescript-fonticon
Finally step, we will need to tell nativescript-fonticon
where to look at, and what is the font icon prefix name so it can do the job of scanning classname and character code for us.
This must be done in app.ts/app.js like below. Notice, this tutorial is for NativeScript-Vue.
import { createApp } from "nativescript-vue";
import Home from "./components/Home.vue";
const app = createApp(Home);
/**
* Font icon
*/
import { FontIconFactory, fonticon } from "nativescript-fonticon";
import { knownFolders } from "@nativescript/core/file-system";
FontIconFactory.debug = true;
FontIconFactory.paths = {
fa: knownFolders.currentApp().getFile("./assets/css/fa.css").readTextSync(),
};
FontIconFactory.loadCss();
// Vue 3
app.config.globalProperties.$fonticon = fonticon;
// Vue 2
// Vue.filter('fonticon', fonticon);
app.start();
Vue 3 has removed filter, so the only way is to define a global function then later use it as this.$fonticon
-> You can change this to other name of your choice.
After that, try ns run
, if you see the console output like this, it scanned sucessfully!
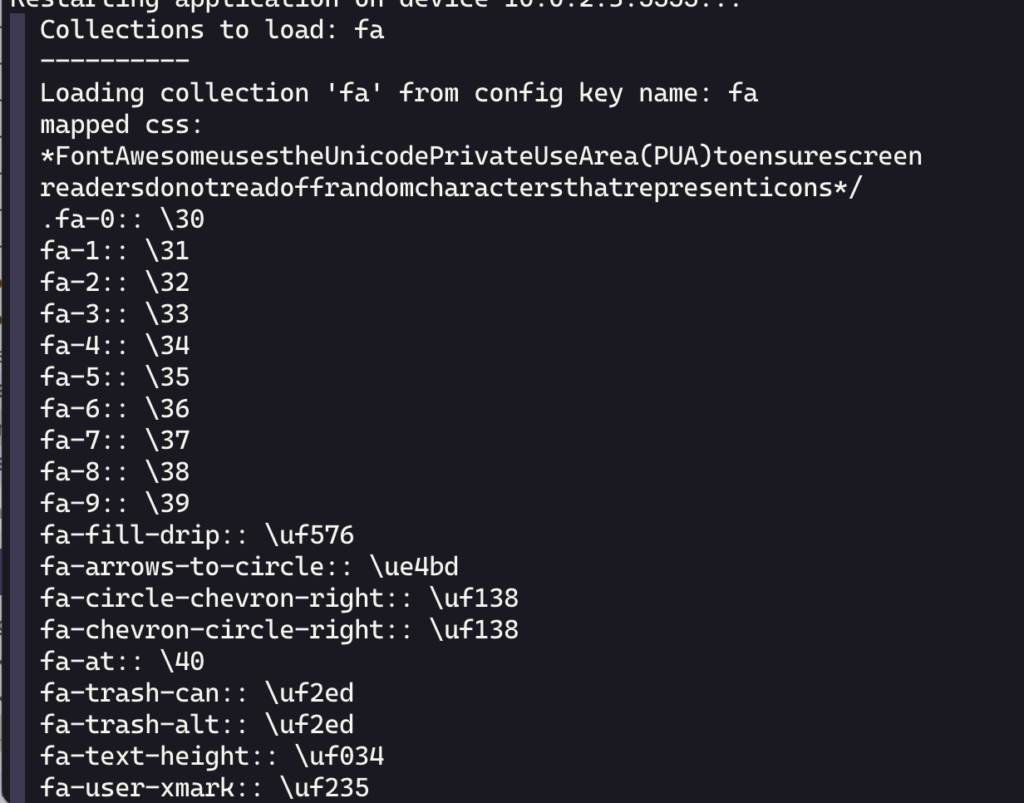
Great, but we may not be able use the icon yet due to a bug in nativescript-fonticon
that prevent it from identify class name properly for FontAwesome. Let’s see how we can workaround this issue.
Head up, ::
vs :
bug in nativescript-fonticon
Updated 2024-05-07: This bug is now addressed in 8.1.0. Make sure you update your package.json to latest version.
If you take a look at FontAwesome provided .css file, you will see each class is defined like this:
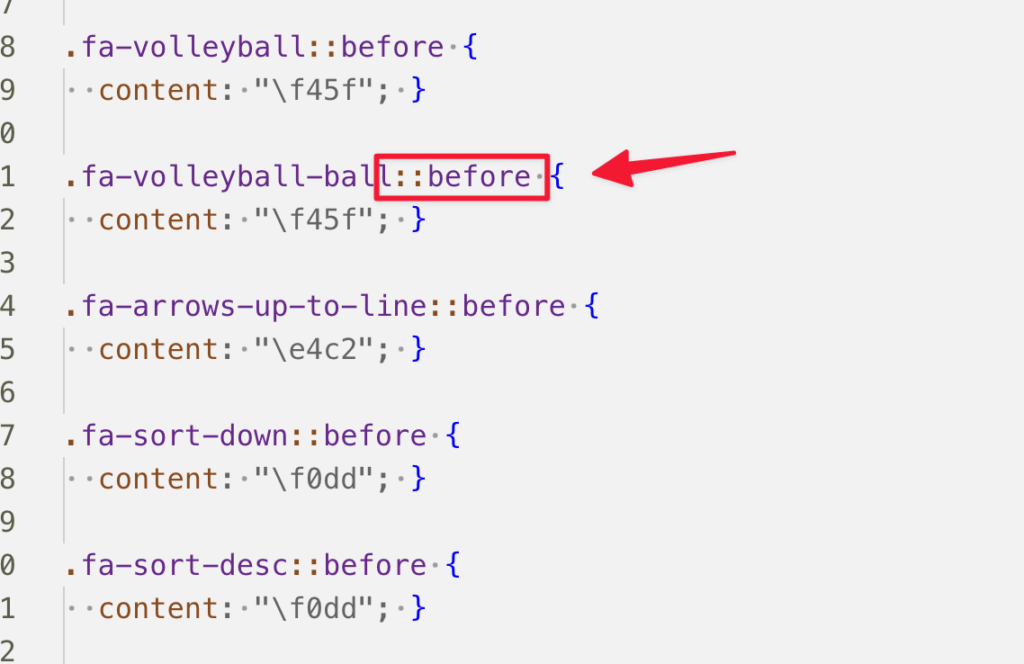
Each icon is defined as:
.fa-volleyball-ball::before {
content: "\f45f"; }
Which use ::before
instead of :before
. While nativescript-fonticon
will only handle :before
(single colon), so it would be the problem. Example if you want to print fa-user
icon, you actually has to use fa-user:
with extra colon after.
If you wonder what is the right standard of using before
pseudo selector, short answer is: ::before
. Long answer, read more at: https://css-tricks.com/to-double-colon-or-not-do-double-colon/
The workaround for this, is replace every ::before
instance in FontAwesome .css with :before
for this setup to work.
In case you are lazy, you can grab mine at: https://github.com/NewbieScripterRepo/PoolPartyFM/blob/part-1-ui/src/assets/fa.css
Same applied to other font icon library until this bug is fixed. I have submitted an PR to nativescript-fonticon here (merged! 🎉) to address this problem. Hope it will get merged soon.
5. Using the icon in your template
Finally, you can now peacefully use the font icon in your template like this:
<Label
class="fas"
:text="$fonticon('fa-explosion')"
/>
For FontAwesome, you can find all icon you can use: https://fontawesome.com/icons . Be aware that you can only use free icon if you don’t have a Pro version. At time time of writting this article, latest FontAwesome is V6.4.2.
And that is all, hope this article help you with installing and using font icon in your NativeScript project. Don’t hesitate to contact via comments below if you experiencing any issues when add font icon to NativeScript, or ping me anytime via NativeScript official discord server, my username is minhnhut. I will try my best to help you if I can. Cheers!